Javascript.Array
An Array object is an indexed, sequenced collection of values, that can themselves be objects, or primitive values like Numbers and Strings. They are more like key-indexed lists than C-language arrays.
construction:
- new Array(initialLength)
- new Array(item1, item2, ...)
- [item1, item2, ...]
attributes:
- length : Integer - (Javascript 1.1, ES 1) a 32-bit integer representing the number of items in the array.
methods:
- pop() : Object or primitive value
- push() : Integer
- reverse()
- shift() : Object or primitive value
- sort() : Array
- splice() : Array
- unshift()
Detailed Method Descriptions
Array.pop() : Object or primitive value
Removes the last element from the array and returns that element to the calling code. If the Array object is empty already, undefined will be returned.
Returns: The element popped of the Array.
Array.prototype.push(item1, item2, ...) : Integer
Adds one or more elements to the end of an array and returns the new length of the array.
Returns: the modified length of the Array.
Array.prototype.reverse()
Reverses the order of the elements of an array in place. The first becomes the last, and the last becomes the first.
Returns: no return value. The elements stored in the Array object are reversed in place.
Array.prototype.shift() : Object or primitive value
Removes the first element from an array and returns that element. This method modifies the length of the Array object.
Returns: the element (an Object or primitive value) shifted off the Array. This object was previously the first item of the Array.
Array.prototype.sort() : Array
Sorts the elements of an array in place and returns the array to the calling code.
Returns: The sorted Array object.
Array.prototype.splice(index, count) : Array
Array.prototype.splice(index, count, extraItem1, ...) : Array
(Javascript 1.2) Adds and/or removes elements from an array. A typical usage would be to remove a certain range of elements within the Array, replacing it with a different chunk. The function returns the removed subset of the Array as a new Array. An empty Array is returned if no objects are removed (unless you are using ancient browser).
parameters:
- index: Integer - the index of the element at which to begin the splice.
- count: Integer - the number of elements to remove. if count is zero, you should specify at least one new element (in the optional parameters to the function)
- extra: Object or primitive value - additional objects to splice into the array in the place of the remove section.
Returns: A new Array object containing as it's elements, the items that were removed from the original Array.
example usage:
var dateComponents = ["YYYY", "MM", "DD"]; var year = "2013"; var month = "07"; var day = "22"; var extracted = dateComponents.splice(0, 1, year); extracted = dateComponents.splice(1, 1, month); extracted = dateComponents.splice(2, 1, day); wisdom.console.log("The date is " + dateComponents[0] + dateComponents[1] + dateComponents[2]);
Array.unshift() : Integer
Adds one or more elements to the front of an array and returns the new length of the array.
Returns: the new length of the array after the unshift operation.
Details
Length
(Javascript 1.1, ES 1) a 32-bit integer representing the number of items in the array. The number always has a positive sign.
The length of an array is the count of it's allocated items. These individual items can be null, undefined, or be object references. An Array object can mutate over time, growing and shrinking as required. Methods are provided to access elements of the array and manipulate them and the array itself.
Setting the length property of an Array object will truncate the Array if it contains more items than the new length value. However, increasing the size of the length property will not create new array element. In this case the length property and the actual number of items in the array become mismatched.
Single-argument Construction:
var myArray = new Array(12);
A single-argument call to the Array constructor initializes the new Array object to a specified length (item count). If this value is an integer between 0 and (2^32 - 1) (inclusive), the resultant JavaScript array has a length of the given number and will contain that many items, each item having the value undefined. This method of calling the constructor can trigger a RangeError exception.
Literal construction:
Array objects can be created using literal initializer lists:
var names = [ "Bob", "Jane", Thabo", "He Who Must Not Be Named" ];
Indexing:
Array objects can be numerically indexed, and this index is zero-based.
var dataPoints = [ 4.5, 55.0, 32.0 ]; // access the 1st item var datum = dataPoints[0]; // access the second item var anotherDatum = dataPoints[1]; // access the last item var lastDatum = dataPoints[2]; // the above can be done more generally like so... lastDatum = dataPoints[dataPoints.length - 1] // ...as long as you know that there is at least one item
Iterating over items in an Array
Here is an example of looping over the values stored in an array in order to print them out to the console:
var animals = ["Dog", "Horse", "Crocodile", "Lion"]; var animalCount = animals.length; for (var i = 0; i < animalCount; i++) { var currentAnimal = animals[i]; wisdom.console.log(currentAnimal); }
Truncating an array (chopping it short)
var planets = ["Mercury", "Venus", "Earth", "Mars", "Jupiter", "Saturn", "Neptune", "Uranus", "Pluto"]; var planetCount = planets.length; wisdom.console.log("The number of planets is " + planetCount); // get rid of Pluto (by new scientific definition of 'planet' planets.length = 8; wisdom.console.log("The number of planets is now " + planetCount);
See also:
This is part of the SpineFORGE Reference Documentation for Javascript?
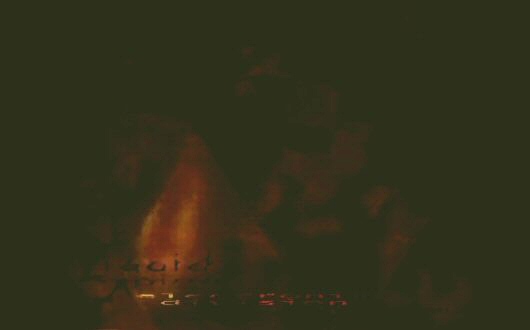