Main.POSIXIteratingThroughADirectory
A function to iterate through a directory on a POSIX system:
Makes use of standard C library, and functions from the POSIX specification (use of dirent.h)
#include <stdio.h> #include <dirent.h> bool listDirectoryContents(const char* path) { struct dirent* entry; DIR* directory; directory = opendir(path); if (directory == NULL) { printf("ERROR: Path does not exist or could not be read.\n"); return false; } while ((entry = readdir(directory))) printf("%s\n", entry->d_name); closedir(directory); return true; }
See also
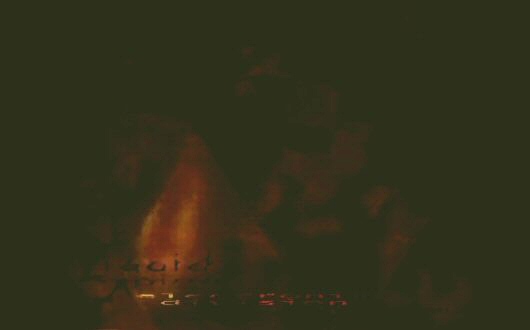