Javascript.Function
In Javascript, functions are first-class objects. They can be created dynamically, and passed around as values.
Here is a small function:
function bark() { alert("Woooof!") }
All the function does it display the string value "Wooof!" in a popup alert box. So our bark() function calls another function, alert().
The token function in the code above declares that a function is to come. Following this, after a space, is the token bark - the name of the function. It's up to you to decide the names of your functions, and this is one of the most difficult parts of the art of programming. Be careful of letting the actual behaviour of your function drift away from the meaning implied by it's name. As your programs mature and increase in size, this can happen more easily than you think .
The next token () made up of round brackets, is the argument list. It being empty in this case flags the function bark as taking no explicit named parameters. This part of a function definition is based on the mathematical concepts and representation of a function, such as the generic format of y = f(x).
On the next line (note that they don't have to be) the curly brackets denote the block of actual code of the function. These lines of code will run when the function is executed. In the bark() example above, the only code inside the function is the single line that calls alert().
The function as written does nothing until it has been "invoked" (or "called"). The code above simply declares and defines the function - it exists now in memory, but needs to be triggered by other external code. Let's try invoke the bark() function:
wisdom.console.log("Invoking the barking function..."); bark(); wisdom.console.log("Returned to calling code.");
Above you can see the invocation of the bark() function, located between two logging calls that print out notices that the function is about to be called, and has returned after (hopefully successful) completion. If you never see the "Returned" notice, you know that something critical did go wrong. You might want to check your browsers' error console for information about the possible error. This "logging-based" method of debugging is simple but effective. The downside is that it can clutter up your code with lots of "narrative", making it hard to see the pattern of the algorithm.
Return Values
Functions can return values (mostly objects or primitive values) to the code that invoked them. Functions that simply perform a specific operation, mutating the global state, or performing some internal calculation could more correctly be called "procedures", as they were in languages like Pascal.
A function can exit from itself, returning a value, using the return statement, providing the possible return value:
function getMeaningOfLife() { ... ... ...difficult calculations... ... ... return 42; } alert("The meaning of life is " + getMeaningOfLife());
There can be multiple return statements scattered throughout a function. If return statements are omitted, then nothing is returned, other than in certain specific conditions. Worthy old lore spake that it is best to fail early and entirely, so this means quitting the function and returning to the calling code (or throwing an Exception) as soon as it can be detected that something is wrong with the function call environment.
- arguments
- arguments.callee
- functions as objects
- options objects as parameters
- Event handlers
See also
Useful wisdom features relating to functions:
This is part of the SpineFORGE Reference Documentation for Javascript?
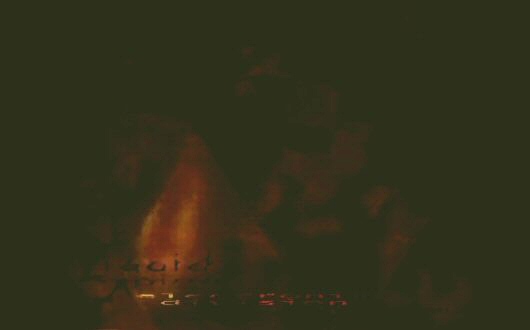