Wisdom.Util
wisdom.util is helper object, which provides some low-level utility infrastructure, features and functionality. Most of these are single-function features too small to deserve dedicated object infrastructure. First-pass feature implementations often begin their lives here, eventually moving to more appropriate modules when they mature or specialize.
found in lib/Util/Util.js
module: wisdom
methods:
methods for Object management:
- discoverProperties(object) : String
- extend(baseObject, object, object, ...) : Object
- isArray(object) : Boolean
methods for Function manipulation:
- argumentsToArray(arg1, ...) : Array
Document-related methods:
Viewport-related methods:
- getViewportWidth() : Integer
- getViewportHeight() : Integer
- getScrollX() : Integer
- getScrollY() : Integer
Element-related methods:
- isDomNode(object) : Boolean
- isDomElement(object) : Boolean
- describeElement(element) : String
- getBodyElement() : Element
- getElement(object | id) : Element
- getElements(id | object, ...) : Array of Element
- getContainerByClassName(element, className1, ...) : Element
- getElementPositionX() : Integer
- getElementPositionY() : Integer
- getElementPosition() : Point2D
Pointer/Mouse methods:
- getDocumentClickX() : Integer
- getDocumentClickY() : Integer
- getMousePositionDocument() : Point2D
- getMousePositionClient() : Point2D
- getMousePositionElement() : Point2D
AJAX methods:
CSS manipulation and rule access:
- hasClass(element, className) : Boolean
- addClass(element, className)
- removeClass(element, className)
- getClasses(element) : Array[String]
- toggleClass(element, classesToRemove, classesToAdd): Boolean
- getCSSRule()
- getComputedStyle(element, stylePropertyName) : String
Event-management methods:
- addEventHandler(element, type, eventHandler)
- getEventType(event) : ? (Text?) or null
- getEventTarget(event) : Element
- getEventObject(event) : Event?
- cancelEvent(event)
Detailed description
Method specifications
wisdom.util.discoverProperties(obj, includeInherited)
Inspect an object, building up a text string displaying the names and types of it's members. It is possible to include inherited properties (those from up the prototype chain). The output is returned as a String.
parameters:
example usage:
var objectDescription = wisdom.util.discoverProperties(someObject); wisdom.console.note(objectDescription);
wisdom.util.isArray(object : Object or primitive value) : Boolean
Returns true if the provided argument is an Array instance, and returns false otherwise.
Implementation notes:
Attempts to make use of one of three methods to discover whether the true type of an object is Array. First the Array.isArray() method is tried, then the instanceof operator, and then using toString().
wisdom.util.extend(baseObject : Object, object : Object, ...) : Object
This function extends a base object (or "default") object with the properties found in objects provided as additional parameters to the function. The first parameter acts as a base object, and if any properties with equivalent names are found in the additional objects that match those in the base object, the final output uses the overridden value.
This function is useful for implementing Options paramters used to construct object instances or configure complicated functions with many parameters.
Returns: a new object, whose attributes are a merging of the attributes of base object and those of the additional objects provided. Default values for attributes are overridden by equivalently-named attributes in the auxilliary parameters.
wisdom.util.argumentsToArray(arg1, ...) : Array
This function is designed as a helper utility for use within methods that need to accept a variable number of parameters, or could gain convenience by accepting either a single Array, or multiple comma-separated arguments.
argumentsToArray accepts either a single Array object, or one or more objects or primitive values. The items stored in the Array, or the separate arguments are repackaged into a Javascript Array and returned to the calling code.
Examples:
Say you have some function you'd like to be able to call like this:
var world = buildWorld(land, sea, air, people); world.name = "Empire"
...but would also like the option to call it like this, depending on the context of the code you might be working on, and the variables and containers you may have access to at the time. Maybe you have an array of things that make up a world:
var worldComponents = [ land, sea, air, people ]; var world = buildWorld(worldComponents); world.name = "Empire"
You want also to be able to use the literal array syntax in place within the function call:
var world = buildWorld([ land, sea, air, people]); world.name = "Empire"
Using this argumentsToArray function, you could implement the buildWorld function like so:
function buildWorld(arg) { var argumentArray = wisdom.util.argumentsToArray(arguments); ... ... // code make use of argumentArray }
wisdom.util.getElements(id : String, ...) : Array[ Element ]
wisdom.util.getElements(element : Element, ...) : Array[ Element ]
wisdom.util.getElements(elements : Array [ String ]) : Array[ Element ]
This function queries the DOM for selections of elements. These elements are requested by making use of selectors, which are either String id's, or are references to the required elements themselves. In future support for CSS-style selectors might be developed.
This method returns an array of references to DOM Elements, that correspond to the provided HTML id's or passed-through element references. If no elements corresponding to the selectors are found, an empty (zero-length) array is returned.
It is possible to alternate the types of values used as query parameters. See example below.
The list of element id's or references can be passed to the function as either a comma-seperated function argument list, or as a single array parameter, which contains the request values.
parameters:
if function argument list, each entry is one of
- id : String HTML id value, or Element reference of required DOM element.
- element : Element reference
if array parameter, a single:
examples:
Retrieve three different elements by explicit, known HTML ids:
var util = wisdom.util; var myElements = util.getElements("ID1", "ID2", "ID3"); // print element ids: for (var i = 0; i < myElements.length; i++) { var currentElement = myElements[i]; wisdom.console.log(currentElement.id); }
the above example could also be expressed like so:
// get the relevant elements: var util = wisdom.util; var e1 = util.getElement("ID1"); var e2 = util.getElement("ID2"); var e3 = util.getElement("ID3"); // print element ids: wisdom.console.log(e1.id); wisdom.console.log(e2.id); wisdom.console.log(e2.id);
...but you can see how that could become unwieldy with many elements. The util.getElement() function as used above is a wrapper for document.getElementById().
To illustrate the possibility of alternate selector types, see this example, which should end up with the same result as the first example:
var util = wisdom.util; var oddElement = document.getElementById("ID2"); var myElements = util.getElements("ID1", oddElement, "ID3"); // print element ids: for (var i = 0; i < myElements.length; i++) { var currentElement = myElements[i]; wisdom.console.log(currentElement.id); }
Notice how oddElement, a previously retrieved DOM element reference, is passed in as a selector. This same element reference will find itself, in place and in order, in the output array returned by this function.
See also getElement()
wisdom.util.getContainerByClassName(element: Element or String id, className, ...) : Element
wisdom.util.getContainerByClassName(element: Element or String id, classNames: Array) : Element
Retrieve a specific ancestor (or container) of an element by providing the element, and a list of class names that must be found on the ancestor/container.
parameters:
- element: Element or String - A DOM reference, or HTML id (provided as a string) to the element to begin the ancestry search. The DOM tree is searched from leaf element towards the root of the tree, checking if each successive ancestor has matching classnames to those provided.
- className1: String holding a single classname which signals the required container, or, if only two arguments are provided to the function, a Array containing a list of classname strings. If the argument list is made up of element, and a comma-separated function argument list of strings, these will be interpreted as the list of classnames to check against.
- classNames: Array - This array contains a collection of Strings, each holding a single class name. The ancestor is expected to match all classes listed here before it is returned.
example:
If you have the following HTML structure, that defines two real-estate properties - my house and it's plot of land, and that of the neighbours:
<!-- my house --> <div class="Courtyard Property" id="theCourtyard"> <div class="Building" id="myHouse"> <div class="Room"> <div class="Table" id="myTable" /> <div class="Chair" id="myChair" /> </div> </div> </div> <!-- neighbours house --> <div class="Courtyard Property"> <div class="Building" id="neighbourHouse"> <div class="Room"> <div class="Table" id="otherTable" /> <div class="Chair" id="otherChair" /> </div> </div> </div>
The following Javascript will find the particular property that currently contains my table:
var util = wisdom.util; var tableElement = util.getElement("myTable"); var tableLocation = util.getContainerByClassName(tableElement, "Building", "Property"); if (tableLocation) alert(tableLocation.id); else alert("This table is lost in the void...");
wisdom.util.getClasses(element: Element) : Array[String]
Return an array of strings representing the one or more class names of the element, if any. Null is returned if the element is invalid, has no classes, or on error.
parameters:
- element: Element - a reference to the element to examine for assigned class names.
returns:
example usage:
var myElement = wisdom.util.getElement("element123"); var classes = wisdom.util.getClasses(myElement); if (classes) { wisdom.console.log("Class List on element:"); var classCount = classes.length; for (var i = 0; i < classCount; i++) { wisdom.console.log(classes[i]); } }
wisdom.util.toggleClass(element: Element | String, classToRemove: String, classToAdd: String)
wisdom.util.toggleClass(element: Element | String, classesToRemove: String | Array, classesToAdd: String | Array)
This function provides for the addition and removal of classnames from Elements.
In it's simplest use, it can swop out one class for another. Other forms allow the removal of one or more elements, while also adding others. Some care is taken to minimize the chance of duplicates, while attempting to keep performance high. Particularly - this function is designed to rewrite the Element.className property in one go, rather than sequentially calling addClass()/removeClass() etc, which might lead to unnecessary page reflows.
parameters:
- element: Either the HTML id (stored as String) of the element required, or a Element reference to the same element.
- classToRemove: A simple text string, with no spaces (ie. a single token), containing the class-name to remove from element, replacing it with the value in classToAdd. See the next entry.
- classToAdd: A simple text string, with no spaces (ie. a single token), containing the class-name to add from element.
- classesToRemove: A simple space-separated text string, each single token containing a class-name to remove from element, replacing it with the values in classesToAdd. See the next entry.
- classesToAdd: A simple space-separated text string, where each single token is a class-name to add to element.
return value: returns true if the function succeeded, false if it fails. If the function generates warnings, it should still run to completion and return true.
wisdom.util.getComputedStyle(element: Element, stylePropertyName: String) : String
Calculate and return the current value of a particular CSS property of an element. The value returned is derived by 'applying the active stylesheets and resolving any basic computation those values may contain.'. The empty string is returned if no APIs can be found to provide the value..
parameters:
- element: Element - a reference to the element which is being queried for it's style property value.
- stylePropertyName: String - the DOM name of the style property to query, as a DOMString value.
returns:
- a String value containing the computed value of the style property of the element.
wisdom.util.addEventHandler(element: Element or String, type: String, eventHandler: Function)
This function registers a new event handling function (an EventListener) against a given type of event, to the provided element. The function eventHandler will be executed when an event of the given type is triggered, either by user interaction, or through some internal application-specific mechanism (a synthetic event).
paramaters:
- element: Element or String - The element upon which to register the new event handling function.
- type: String - the type of event to register the event handling function. See the list of valid values below.
- eventHandler: Function - A function object, the function that will be called when the specified event is triggered on the element.
Possible values for the type parameter are dependant on the element type. Here are some examples:
- "click"
- "hover"
- "mouseover"
- "mouseout"
- "mousedown"
- "mouseup"
- "load"
- "resize"
See also
This is part of the Reference Documentation for the Wisdom Javascript Library? (or wisdom.js)
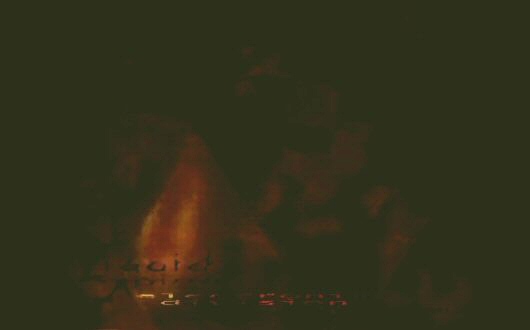