Wisdom.SlidingPanel
A SlidingPanel is a dynamic, 2D, box-like frame for presenting multiple sub-"panes" of content, which are viewed through a viewport-like area of the containing web page. The main sliding panel, which contains the individual panes, sides in one or more directions based on user input or scripted events. Various options are available for controlling the behaviour and presentation of the content.
found in: lib/SlidingPanel/SlidingPanel.js
dependancies:
constructor:
- wisdom.ui.SlidingPanel(options : Options)
constructor options:
- element : String or Element - Id of element, or element reference of the DOM element to be used as the main sliding panel. This element should be contained by the viewport: an element of class WisdomSlidingPanelViewport. This is the element that contains the individual panes (it is not the viewport through which the panes are viewed).
- direction : Flag - One of :
- wisdom.ui.SlidingPanel.Vertical
- wisdom.ui.SlidingPanel.Horizontal
- manual : Boolean - Slide only on user interaction (eg button click). See the notes for options.prevButtonId and options.nextButtonId.
- loop : Boolean - Whether or not to loop back to the starting view when the sliding panel reaches reaches it's end position. This value can be used whether or not options.manual is true.
- frameInterval : Number - The number of millieconds per frame (or "tick") (This is equivalent to 1/FPS, where FPS is "desired frames-per-second"). The browser will attempt to update the SlidingPanel every frameInterval milliseconds. If the update activity takes too long, the interval may end up larger than requested.
- interpolation (Flag) : The interpolation type. One of:
- wisdom.ui.SlidingPanel.InterpolationLinear
- wisdom.ui.SlidingPanel.InterpolationExponential
- interpolationFactor Number : If interplation has the value wisdom.ui.SlidingPanel.InterpolationExponential, this value can contain a divisor value for the movement interpolation. Larger numbers make for slower animations. Default is 2. A value of 2 or more is recommended. A value of exactly 1 will cause instantaneous transitions.
- frameMoveDistance : Number - When interpolation is set to wisdom.ui.SlidingPanel.InterpolationLinear, this value controls the distance a SlidingPanel moves per frame/tick (ie. every frameInterval ms)
- pauseInterval : Number - The number of milliseconds that the SlidingPanel will pause on an item before transitioning to the next. This option only really makes sense when options.manual is false (ie the SlidingPanel is animating automatically without user input).
- viewportWidth : Number - The width of the viewport in CSS pixels (required if SlidingPanel.Horizontal). See the notes on sizing SlidingPanels below.
- viewportHeight : Number - The width of the viewport in CSS pixels (required if SlidingPanel.Vertical). See the notes on sizing SlidingPanels below.
- itemCount : Number - The number of items/panes in the SlidingPanel
- itemWidth : Number - The width of each sliding item in the SlidingPanel, in CSS pixels (required if SlidingPanel.Horizontal). Not required if autoSize is true. See the notes on sizing SlidingPanels below.
- itemHeight : Number - The height of each sliding item in the SlidingPanel, in CSS pixels (required if SlidingPanel.Vertical). Not required if autoSize is true. See the notes on sizing SlidingPanels below.
- autoSize: Boolean - If true, figure out pane sizes automatically rather than use the itemWidth/Height fields. If autoSize is provided, you do not need to provide itemWidth or itemHeight parameters. See the notes on sizing SlidingPanels below.
- viewableItems: Integer - If zero, used provided fixed widths and heights, otherwise, make sure the viewport is proportioned to show this many panes at once. See the notes on sizing SlidingPanels below.
- fadeOnCycle : Boolean - Whether or not to fade on cycle (only really works for long lists)
- fadeOnFocus : Boolean - Whether or not to fade in the current target pane, and fade out all others. Fading is calculated based on distance from viewport origin.
- alertOnErrors : Boolean - When this value is true, any error generated by the SlidingPanel module will cause an alert box to pop up, displaying the error message to the user, instead of logging the issue to wisdom.console.
- resetItemCount : Integer - A (potentially negative) integer used to control the cycling/reset of the items (ie. This is an offset on the itemCount used to calculate total movement distance). Default 0. This value is often useful to have set to 1, so that when looping around on itself, the last pane can smoothly exit the viewport before the panels reset to their starting position again. NOTE: Currently using a value > 0 for resetItemCount when options.manual is true will cause the user to manually have to bypass the last "extra" panes, which may well be totally devoid of content.
- scrollItemCount : Integer - An integer controlling how many items/panes must scroll past before pausing. (ie. A number of items to skip before pausing). This presumes that the pausing behaviour is in use. Default 1.
- prevButtonId : String - Id of the element to be used as the "previous button" which will trigger a switch to the previous pane. The given element will be assigned an onclick event handler that triggers the transition to the previous pane or position.
- nextButtonId : String - Id of the element to be used as the "next button" which will trigger a switch to the next pane. The given element will be assigned an onclick event handler that triggers the transition to the next pane or position.
- updateCallback : Function - A function that will be called every update tick (used for customization). This callback will receive the SlidingPanel object as a parameter
methods:
- go(paneIndex : Integer) : Transition the view to another pane. The pane is specified by index. The current interpolation type will be used to animate the movement.
- getPanelWidth() : Integer) : Return the total width of the SlidingPanel (total of all pane widths).
Detailed information
SlidingPanels can be used to contain a number of "panes" stacked vertically or horizontally, that can be navigated by sliding them horizontally or vertically within a "viewport"-like containing frame (which you might want to augment with a wisdom.ui.PresentationFrame). Additionally SlidingPanels can be used to display lists of information or images such as in breaking news "ticker-tape"-style interfaces.
The sliding behaviour of a SlidingPanel can be triggered by user input (using DOM elements such as clickable buttons, hover-enabled triggers, or some kind of directional interface), or left to animate by itself following a timed sequence (which is the default). In addition, multiple movement modes are available which control the motion of the panes as they swing into and out of view.
Usage example:
Here is an example of integrating a SlidingPanel and it's content pages into an HTML page.
In this example a set of 4 content panes (those elements given the class "WisdomSlidingPanelPane") are placed inside a parent element which will act as the main SlidingPanel ( which itself has been given the class "WisdomSlidingPanelHorizontal"). This SlidingPanel containing the four panes will slide horizontally inside the viewport created by the element with class "WisdomSlidingPanelViewport".
The 4 panes and the viewport must have widths and heights that match the equivalent parameters passed to the wisdom.ui.SlidingPanel() constructor function (options.itemWidth/Height and options.viewportWidth/Height). These dimensions can be specified by inline CSS styles or as class overrides in an application-specific CSS file or style tag.
<h1>Demo: wisdom.ui.SlidingPanel</h1> <h2>Welcome to this Wisdom Demo Page!</h2> <p>This is a test page for the wisdom.ui.SlidingPanel user-interface component</p> <div id="slidingPanelViewport" class="WisdomSlidingPanelViewport"> <div id="slidingPanel" class="WisdomSlidingPanelHorizontal"> <div id="pane1" class="WisdomSlidingPanelPane"> <h2>Pane 1</h2> <p>Some content</p> </div> <div id="pane2" class="WisdomSlidingPanelPane"> <h2>Pane 2</h2> <p>Some content</p> </div> <div id="pane3" class="WisdomSlidingPanelPane"> <h2>Pane 3</h2> <p>Some content</p> </div> <div id="pane4" class="WisdomSlidingPanelPane"> <h2>Pane 4</h2> <p>Some content</p> </div> </div> </div> <input id="prevPage" name="prevPageButton" type="button" value="Prev"> <input id="nextPage" name="nextPageButton" type="button" value="Next"> <p id="mySprite">Hello this is cool!</p> <div id="wisdomConsole" class="WisdomLog" /> <!-- Include wisdom library --> <script type="text/javascript" src="../../wisdom_release_min-0.3.js"></script> <script> // Your application code, or links to other scripts wisdom.console.log("Hello"); var slidingPanel = new wisdom.ui.SlidingPanel({ element: "slidingPanel", prevButtonId: "prevPage", nextButtonId: "nextPage", direction: wisdom.ui.SlidingPanel.Horizontal, manual: true, loop: true, interpolation: wisdom.ui.SlidingPanel.InterpolationExponential, interpolationFactor: 5, itemCount: 4, itemWidth: 640, itemHeight: 480, frameInterval: 30, frameMoveDistance: 20, viewportWidth: 640, viewportHeight: 480 /* Only really need width when making horizontal sliding panels */ }); </script>
This is part of the Reference Documentation for the Wisdom Javascript Library? (or wisdom.js)
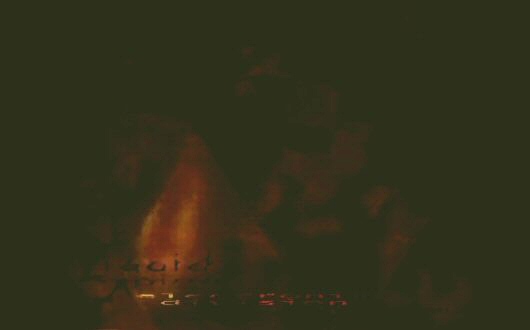