Wisdom.Tooltip
A Tooltip is a small panel of content that appears when an assigned element in a document is "hovered" over by the user's pointer (or mouse cursor). They usually contain hints and tips about the application function the user intends to activate, disappearing when the user moves the pointer away from the triggering element. The Tooltip can appear by simply popping into view, or fading in and out.
Wisdom Tooltips are implemented by creating a <DIV> element within the document that will acts as the content of the tooldtip when it appears. It is given an id attribute and a specific CSS class, along with the element that must trigger it's appearance. A snippet of Javascript on the page links the triggering element to the tootip, allowing it to behave as it should. Once the tooltip has been created, it can be forgotten. The CSS classes applied to the Tooltip element help provide it's show/hide functionality.
found in: lib/Tooltip/Tooltip.js
depedancies:
constructor:
- wisdom.ui.Tooltip(contentElementId: String, tooltipElementId: String, options: Options)
- wisdom.ui.Tooltip(options: Options)
In the first form of the constructor (with three arguments), the first two actual arguments provided must be strings, containing the relevant HTML id values of the elements required.
In the second form of the constructor, an options object is provided, whose members should contain the following values:
- contentElement: an HTML id as a String, or a DOM Element reference to the element that will trigger the tooltip.
- tooltipElement: an HTML id as a String, or a DOM Element reference to the element that will act as the tooltip. The contents of this element will be displayed when the tooltip becomes visible.
- maxOpacity: Number - a floating-point value between 0.0 and 1.0. The maximum opacity that a fading tooltip will reach. A value of 0.85 or 0.9 will provide a nice, some-what transparent effect.
Detailed Information
In the simplest scenario, you have one or more items in your document that require triggering popup panels that hover near the element or cursor/pointer, providing some useful information to the user, and these then disappear when the pointer is removed from the element. The content of the tooltips is static and pre-defined. In this case, all that's needed is to construct the tooltip content containers (usually DIV elements), give them the requisite wisdom CSS classes, fill them with the images, icons and text you require, and using a snippet of Javascript, construct the wisdom.ui.Tooltip object, providing references or id's of the elements set aside for the purpose (this usually happens in the tail-end of the page, or via the onload document event.
For each element that should pop up a tooltip, you need a collaborating tooltip container element that exists from the beginning of page load/display, but is hidden by default. The content that triggers the tooltip is given an id, as is the tooltip element itself.
It is possible to create dynamic tooltips that change depending on application-specific context by modifying the contents of the tooltipElement using other code, just before the tooltips are triggered (or all the time, in case they are triggered).
Another technique would be to create a single tooltip element on the page that is re-used for each occurance of a tooltip popping up, regardless of the element that triggered it. Your code could modify this single "global" tooltip each time is is displayed, making decisions about what content to place in the tooltip based on the content element id, or maybe the content itself - think about a dictionary application that could describe a word in a paragraph, or a translator, that popped up a translated version of a paragraph.
Example Usage
Here is a small test of the tooltip (complete HTML):
<html> <head> <link rel="stylesheet" type="text/css" href="wisdom_release_style.css"/> <script type="text/javascript" src="wisdom_release_min.js"> </script> </head> <body> <h1 class="MainHeading">A Tooltip Test</h1> <p>There's things to say and things to be said, more than that, there's things to be told...</p> <p id="mainPara">This is a random paragraph of non-interesting text, containing some words, and punctuation. </p> <p>Here is yet another terribly written paragraph containing no real wisdom or wit whatsoever.</p> <div> <p>This particular piece of lore has been forgotten...</p> <p id="mainHeading1"> ...and this written piece is devoid of meaning or importance </p> </div> <div class="ToolTip" id="tooltip1"> <h1>Remember this name!</h1> <p>you will regret it if you don't.</p> <div class="StarIcon" width="10px" height="10px"> * </div> </div> <div class="ToolTip" id="tooltip2"> <h1>A second tooltip</h1> <p>containing some text</p> <div class="StarIcon" width="10px" height="10px"> * </div> </div> </body> <script> var mainHeadingToolTip = new wisdom.ui.ToolTip("mainHeading1", "tooltip1", { maxOpacity: 0.85 }); var tooltip2 = new wisdom.ui.ToolTip ({ contentElement: "mainPara", tooltipElement: "tooltip2", maxOpacity: 0.4 }); </script> </html>
See also
This is part of the Reference Documentation for the Wisdom Javascript Library? (or wisdom.js)
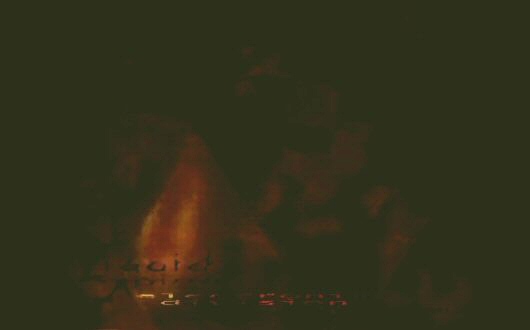