Wisdom.Sprite
Sprites are moveable, sizeable visual elements that can be used as building-blocks for more complex application widgets or animated images.
found in /lib/Sprite/Sprite.js
dependencies:
constructor:
- wisdom.ui.Sprite(options : Options)
- wisdom.ui.Sprite(containingScene : Element|String, useContainerAsElement : Boolean) (deprecated)
properties:
- element : Element (ro)
- container : Element (ro)
- logging : Boolean
- deferGeometryChanges : Boolean
- positionContainer : Boolean
- x : Number (ro)
- y : Number (ro)
- z : Number (ro)
- width : Number (ro)
- height : Number (ro)
- opacity : Number (ro)
- useFilterAlpha : Boolean
functions:
- absolute()
- fixed()
- relative()
- position(x,y,z)
- position(x,y)
- position(newPosition : wisdom.geometry.Point3D)
- position2D(x,y)
- position3D(x,y,z)
- size(x,y)
- size(wisdom.geometry.Point3D)
- show()
- hide()
- visible()
- invisible()
- applyGeometryChanges()
- setOpacity(opacityValue)
- color(cssValue)
- colorRGB(r,g,b)
- colorRGBA(r,g,b,a)
- colorRGBFloat(r,g,b)
- colorRGBAFloat(r,g,b,a)
- backgroundColor(cssValue)
- backgroundColorRGB(r,g,b)
- useBackgroundImage(Javascript/String url)
- backgroundImageUrl() : String
- useImage(url : String)
- imageUrl() : String
- useEventHandler(eventType : String, eventHandler : Function);
event handling:
- onload()
- onclick()
- onmouseover()
- onmouseout()
- onmousedown()
- onmouseup()
- onresize()
- onunload()
Usage information
A Sprite is a rectangular screen area used to display graphics that can move and resize. If more advanced browser features are available, it can also fade in and out, and display animated imagery within.
A Sprite can be made of existing elements, or created outside of the document tree and inserted somewhere later. The most important properties of a Sprite are it's element, and it's container. The element is the physical embodiment of the Sprite, while the container is the scene upon which the Sprite is positioned and animated.
There are many ways to build a Sprite: the DOM element that will act as the canvas or scene upon which the sprite's embodiment will move can be provided to the Sprite() constructor function, or a direct reference (via DOM reference or text ID) to a pre-existing HTML element to use as the Sprite element itself can be provided.
Once in the document tree, a Sprite can be moved around and resized, shown or hidden. This is done generally within the area of the Sprite's containing element (such as a div). In order to get the Sprite's positioning working properly, the Sprites' container must be "positioned" (ie have a width and height, and not be statically placed in the document flow).
A Sprite can contain an <IMG> element in order to display an image, or it can use a CSS background image to achieve the same thing (although different limitations, pros and cons exist either way). You can dynamically change the image while it is displaying simply by changing it's image URL.
Create a Sprite by calling the wisdom.ui.Sprite() constructor function. When called with no parameters the sprite will create an extra-document element for itself and apply it's default properties. You can pass a javascript object containing a reference to a DOM node that will act as the containing scene for the Sprite - typically this is a large block region that fills the browser window.
var sceneDiv = document.getElementById("myscenediv"); var mysprite = new wisdom.ui.Sprite(sceneDiv); mysprite.size(32,32); mysprite.position(100,180); mysprite.useImage("images/particles/sparkle1.png"); mysprite.useEventHandler("click", clickParticle);
... where clickParticle is defined like this:
var scene.clickParticle = function(event) { alert("Poof"); }
Sprites generally behave as absolutely-positioned block elements with explicit heights and widths, that move relative to their containing "scene" element. Absolute positioning means they act outside of the general flow of other child elements of the containing scene element.
You can use a background image or a proper <img> element to display the sprite graphics. You can also use both at the same time. Browsers that support alpha transparency will correctly use this property of the source images. Note that you cannot resize a background image with your sprite element. If you need to dynamically scale your sprite, use an image instead.
If you require images or background images with alpha-transparency that work in IE6, do not use the functions useImage() or useBackgroundImage() to do the actual application of the image to the Sprite, but rather create the Sprite object and set it's element id to one that has predefined CSS rules for background-image and the hackish DX filters. See Sprite::useImage() for more details
You can also use animated gif images for amazing effects with moving Sprites in browsers that support them.
Sprite objects will attempt to use what structures are made available to them.
Constructor Documentation
There are two methods of constructing a Sprite object. The second is deprecated.
Preferred construction method:
This constructor makes use of an "options object", a simple Javascript object who's named properties represent parameters or values used to initialize the Sprite. The options used here largely echo the attribute and function lists of the Sprite itself.
element
Either a String containing the ID of, or Javascript object-reference to, the DOM node to use as the Sprite's visual representation. An object-reference value can be retrieved using document.getElementById(). If element is specified it is given priority as primary DOM representation for the sprite. If options.element is null or missing, then the sprite is initialized as a "free" Sprite object, unattached to the DOM, regardless of any container value, if any. If a container is also provided, and element is a text String, then the value of element will be used for the new Sprite's element id, unless it corresponds to an existing identified element, in which case checks for correct parent relationships will be performed. If the actual DOM structure does not mirror the requirements for the sprite's insertion into the DOM, the construction will fail. In other words, if both container and element are references to valid DOM objects or ID's thereof, they must have a corresponding parent-child relationship in the DOM. No re-parenting or restructuring of the DOM will be performed. If a valid container is provided (either as an existing text id or DOM node reference), but element is not, then a new DOM element will be created during the construction of the Sprite object and be appended as a child of the given container node. The new Sprite element will have no explicit id (though one can be set later, or a value provided for newElementId). Unless the className attribute is provided, the new sprite's element will have the the default CSS class 'Sprite' added to it's Element.class attribute value.
container
Either a String containing the ID of, or a Javascript object-reference (such as retrieved using document.getElementById()) to, the DOM node to use as the Sprite's containing "scene" or "stage". This is the element that (barring special tricks) the child Sprite element will move around on. If a container is provided (either as an existing text id or node reference), but element is not, the sprite will take care of the creation of it's own visual element within the DOM: a new DOM element will be created during the construction of the Sprite object and be appended as a child of the given container node. The new Sprite element will have no explicit id unless one is set later or a value provided for the newElementId property. See the documentation for the element option above for more details on the relationship between and Sprite element and container.
className
Unless this property is provided, the sprite element will have the the CSS class 'Sprite' added to it's Element.class attribute value. This property therefore acts as a potential override for the default wisdom Sprite CSS rules.
Deprecated pre-0.2 method:
blah blah
Function Documentation
absolute()
Set the Sprite element to use CSS absolute positioning.
fixed()
Set the Sprite element to use CSS fixed positioning.
relative()
Set the Sprite element to use CSS relative positioning.
position(x : Number, y : Number, z : Number)
Change the position of the Sprite, with positions given as floating-point Numbers? on the x,y and z axes. The z value controls the CSS z-index value of the Sprite element. If deferGeometryChanges is false the actual CSS style will not be set - only the Sprite object's internal properties will reflect the change, until the updateGeometry() method is called, at which point a page re-flow might occur.
position(x : Number, y : Number)
Change the position of the Sprite, with positions given as floating-point Number values on the x and y axes, with z (the CSS z-index of the element) being left as it was before the function was called. If the Sprite's deferGeometryChanges attribute is false the actual CSS style will not be set - only the Sprite object's internal properties will reflect the change until the updateGeometry() method is called, at which point a page re-flow might occur.
position(newPosition : wisdom.geometry.Point3D)
Change the position of the Sprite, with the new position provided as a Point3D object. If the Sprite's deferGeometryChanges attribute is false the actual CSS style will not be modified - only the Sprite object's internal properties will reflect the change until the updateGeometry() method is called, at which point a page re-flow might occur.
position2D(x : Number, y : Number)
Change the position of the Sprite on the x and y axes only, with positions given as floating-point Number? values. The new position are provided as 2 Numbers. If the Sprite's deferGeometryChanges attribute is false the actual CSS style will not be modified - only the Sprite object's internal properties will reflect the change until the updateGeometry() method is called, at which point a page re-flow might occur.
position3D(x : Number, y : Number, z : Number)
Change the position of the Sprite on the x, y and z axes. The new position is provided as 3 floating-point Numbers. If the Sprite object's deferGeometryChanges attribute is false the actual element's CSS style will not be modified - only the Sprite object's internal properties will reflect the change until the updateGeometry() method is called, at which point a page re-flow might occur.
Resize the Sprite object by modifying it's height and width. If the Sprite's deferGeometryChanges attribute is false the Sprite element's actual CSS width and height will not be modified - only the Sprite object's internal properties will reflect the change until the updateGeometry() method is called, at which point a page re-flow might occur.
size(newSize : wisdom.geometry.Point3D)
Resize the Sprite object by modifying it's height and width, with the new size provided as a Point3D object. The z axis value is ignored. If the Sprite's deferGeometryChanges attribute is false the Sprite element's actual CSS width and height values will not be modified - only the Sprite object's internal properties will reflect the change until the updateGeometry() method is called, at which point a page reflow might occur.
show()
Show the Sprite by modifying the CSS display property of it's element to "block". The effect is immediate (ie. deferGeometryChanges() has no effect).
hide()
Hide the Sprite by modifying the CSS display property of it's element to "none". The effect is immediate (ie. deferGeometryChanges() has no effect). When hidden in this way (as opposed to using the invisible() function, the element takes up no space in the document and does not affect elements around it.
visible()
Show the Sprite by modifying the CSS visibility property of it's element to "visible". The effect is immediate (deferGeometryChanges has no effect).
invisible()
Hide the Sprite by modifying the CSS visibility property of it's element to "invisible". The effect is immediate (deferGeometryChanges has no effect). When hidden in this way (as opposed to using the hide() function, the element still takes up space in the document and affects the elements around it as though it was still there (which it is).
applyGeometryChanges()
Apply any changes made to the Sprite's internal properties such as position and size, to the DOM, most likely causing page re-flow.
setOpacity(opacityValue : Number)
Set the opacity Sprite content area and contents using a normalized floating-point value (ranging from 0.0 to 1.0). Out of range values will be clamped.
Examples: // half-tansparent: s.setOpacity(0.5); // full opaque (not see-through): s.setOpacity(1.0);
TODO: Set to display:none if full-transparent etc.?
color(cssValue : String, <optional> opacity : Number)
Set the foreground color of the Sprite contents. The cssValue argument is presumed to be a text string containing a complete CSS colour specification such as "red" or "#00AA00" (representing green). The opacity parameter is optional and if given, must be a normalized floating point Number value (range 0.0 - 1.0). Out of range values will be clamped.
Examples: // half-transparent red: s.color("#FF0000", 0.5); // fully-opaque green: s.color("#00FF00", 1.0); // blue (opacity left as previous to call): s.color("#0000FF"); // green-grey (opacity left as previous to call): s.color("#AABBAA");
colorRGB(r : Integer, g : Integer ,b : Integer)
Set the foreground color of the Sprite contents using Integer RGB values in a byte range of 0-255 each for red, green, and blue colour components. Opacity is left at whatever is was set to before this method is called. Out of range colour values are clamped.
Examples: // bright red s.colorRGB(255, 0, 0); // dark-green: s.colorRGB(0, 160, 0); // blue: s.colorRGB(0,0,255);
colorRGBA(r : Integer, g : Integer, b : Integer, a : Number)
Set the foreground color of the Sprite contents using Integer RGB values in a 0-255 byte range for red, green, and blue colour components), as well as a normalized floating-point opacity value. If the final opacity term is left out, opacity is left untouched. Out of range colour values are clamped.
Examples: // bright red, half-transparent: s.colorRGBA(255, 0, 0, 0.5); // dark-green, fully-opaque: s.colorRGBA(0, 160, 0, 1.0); // blue (opacity left as previous to call): s.colorRGBA(0,0,255);
colorRGBFloat(r : Number, g : Number, b : Number)
Set the foreground color of the Sprite contents using normalized floating-point numeric values (0-1.0 range for red, green, and blue). Opacity is left as it was pre-call. Out of range colour values are clamped.
Examples: //Red: s.colorRGBFloat(1.0, 0, 0); // Dark-green: s.colorRGBFloat(0.0, 0.6, 0.0); // Blue: s.colorRGBFloat(0,0,1);
colorRGBAFloat(r : Number,g : Number, b : Number, a : Number)
Set the foreground color of the Sprite contents using normalized floating-point numeric values (0-1.0 range for red, green, and blue, and opacity). If the final opacity term is left out, opacity is left as it was pre-call. Out of range colour or opacity values are clamped.
Examples: // half-transparent red: s.colorRGBAFloat(1.0, 0, 0, 0.5); // fully-opaque dark-green: s.colorRGBAFloat(0.0, 0.6, 0.0, 1.0); // blue (opacity left as previous to call): s.colorRGBAFloat(0,0,1);
backgroundColor(cssValue : String)
Set the background color of the Sprite drawing area. The cssValue argument is presumed to be a text string containing a complete CSS colour specification such as "black" or "#FFFFFF" (which represents white).
Examples: // bright red: s.backgroundColor("#FF0000"); // bright green: s.backgroundColor("#00FF00"); // dark blue: s.backgroundColor("#000066"); // green-grey: s.backgroundColor("#AABBAA");
backgroundColorRGB(r : Integer, g : Integer, b : Integer)
Set the background color of the Sprite contents using integer RGB values (0-255 byte range for red, green, and blue).
Examples: // bright red: s.backgroundColorRGB(255, 0, 0); // dark-green: s.backgroundColorRGB(0, 160, 0); // blue: s.backgroundColorRGB(0,0,255);
setOpacity(opacityValue : Number)
Set the opacity Sprite content area and contents using/ a normalized floating-point value (ranging from 0.0 to 1.0)
Examples: // half-tansparent: s.setOpacity(0.5); // full opaque (not see-through): s.setOpacity(1.0);
TODO: Set to display:none if full-transparent etc.?
useBackgroundImage(url : String)
Set the background image of the Sprite element by providing an image URL.
backgroundImageUrl() : String
Return the current Sprite background image URL.
useImage(url : String)
Use a given image for the sprite as an image element, rather than a style-based background image. The width, height and altText are optional parameters.
Limitations of image use:
If the image contains transparency (PNG-style) and you need the effect to be visible on IE6, you need:
A) to set the Sprite,useFilterAlpha property to true before calling this function.
B) you should create the Sprite from an existing document element, rather than from it's container element (See the Sprite() constructor). C) The sprite element needs to have CSS rules applying a filter to it. This is best achieved by having these rules existing in your conditionally-included "IE-hacks" stylesheet as a static rule, which can then be overridden later by Javascript. If the rules are not specified statically in the stylesheet, this function will fail to detect filters on the element and the usual image loading functionality will happen instead.
imageUrl() : String
Return the URL of the image used for the Sprite.
useEventHandler(eventType : String, eventHandler : Function)
Set up an event handler function for a specific named event.
Possible events:
- "click"
- "dblclick"
- "mouseover"
- "mouseout"
- "mousemove"
Detailed Tutorials
TODO: Usage types (mass-anonymous such as particle engines, or controllable unique widget)
Create a new Sprite object by calling the Sprite() constructor function. This sprite is not yet part of the document tree. It could be called a 'free sprite', as yet mostly unconfigured. It references new free element, with it's className set to 'Sprite'
var s = new Sprite();
Use an existing page element as the Sprite element
var element = document.getElementById("something"); var s = new Sprite(element);
or
var s = new Sprite(document.getElementById("something"));
When given no parameters, the Sprite object is created without an explicit scene element to use as its' containing block or as a physical element. It is not attached to the document tree. It is possible to attach the Sprite object to a document element and become it's controller later on.
var s = new Sprite(); var e = document.getElementById("myspritenode"); s.useElement(e);
When given ONE parameter, the parameter is first assumed to be a reference to the actual node to be used as the physical sprite element, which moves about within it's containing block
var elem = document.getElementById("myspritenode"); var s = new Sprite(elem);
or, if the single parameter is a text string, it is assumed to be the id of the element to use as the sprites' physical element:
var s = new Sprite("mysprite");
also, if the single parameter is an object, rather than an element reference or text id, it is treated as an "options" object, that contains properties applicable to the Sprite being created:
var s = new Sprite( {element: document.getElementById("monster1") });
If more than two parameters are provided to the Sprite constructor, only the first 2 are examined. In this case, the first parameter is presumed to be the reference to an element to use as the sprites' embodiment, or the text id of the element to use.
var s = new Sprite("sprite1234", { width: 32, height: 32 });
It is then possible to further specify interesting behaviours of the Sprite using this initializer or "options" object:
var s = new Sprite("sprite1234", { container: "scenediv", color: "red", width: 32, height: 32 });
There are many ways to format the options object. Here is it presented on one line, nice and short:
var s = new Sprite( { container: "mySceneDiv", image: "images/monster.jpg" } );
or potentially more readably across multiple lines:
var s = new Sprite({ container: "mySceneDiv", image: "images/monster.jpg" });
In an ideal world of compatible browsers we could create a sprite than can move over the entire document like so:
var s = new Sprite(); s.useContainer(document.body);
or another way to achieve the same thing:
var s = new Sprite({container: document.body });
But this is better done using a utility function that wraps the various browser inconsistancies: var s = new Sprite();
s.useContainer(getDocumentElement());
Specify (during creation) an existing page element as the container which acts as the 'containing block' or 'stage') for the Sprite:
var s = new Sprite( { container: "MyId345" } );
Or to belatedly use a certain element as it's containing block some time after creation:
var s = new Sprite(); ... var e = document.getElementById("myscenediv"); s.useContainer(e);
Note that in these cases, container is ignored if any text id given refers to an actual indentified HTML element):
var s = new Sprite( "MyId342", { container: "MyId345" } ); var s2 = new Sprite( { element: "logo", container: "MyId347" } ); var s3 = new Sprite( someElement, { container: "MyId347" } );
Note that in s2 below, we override options.container by giving an actual element reference:
var options = { container: document.body, element: "node13" } var s = new Sprite(options); var s2 = new Sprite("MyId23", options);
This is part of the Reference Documentation for the Wisdom Javascript Library? (or wisdom.js)
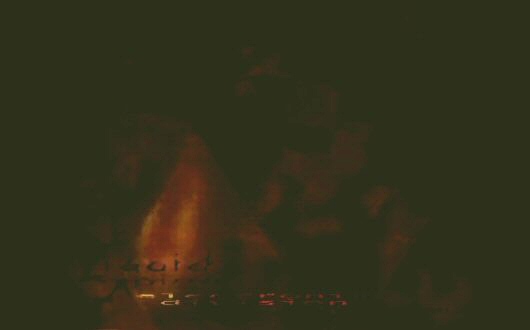